Ticket Master System Design
Never dive directly into the design phase; it may raise red flags during the interview!
Interviewer: We need a system where users can buy tickets for events like concerts and sports. It should handle high traffic smoothly, especially during big events.
1. Feature Expectations [5 mins]
You: Could you clarify a few things before we start working on the design? More specifically, how many events at once do we anticipate managing? Do we also need to allow for real-time ticket availability updates?
Interviewer: We expect to handle hundreds of events at the same time, especially during peak seasons. Real-time updates for ticket availability are crucial to ensure users see accurate information.
You: Thank you for the insights. This helps in shaping the design. I’ll proceed considering these factors.
Functional Requirements
- Select and Purchase Tickets: Users should be able to select tickets for an event, choose their preferred seating or ticket type, and complete the purchase.
- Search and Browse Events: Users should be able to search for and browse available events by category, location, and date.
- View Event Details: The system should display real-time updates on ticket availability, ensuring users see the most current information and preventing overselling of tickets.
The core features we’re focusing on are crucial to how users engage with the Ticket Master platform. At this stage, we won’t address secondary features like in-depth analytics or advanced user profiles, though these are also important.
Understanding the different user types is essential. We cater to event-goers searching for tickets, event organizers handling ticket sales, and venue managers coordinating seating. Each user group has unique requirements, from simple ticket purchases to detailed event management.
By recognizing whether our users are attendees, organizers, or venue operators, we can tailor the platform to better serve their needs and enhance their overall experience.
Limit the number of features discussed to one or two, as covering more can be time-consuming and may detract from explaining the most critical aspects of the design.
2. Estimations [5 mins]
Estimations are essential in the system design of a ticket management system as they help predict the required resources, such as network bandwidth, storage, and processing power, to handle expected ticket volumes. Accurate estimations ensure the system is designed to meet demand efficiently, preventing bottlenecks and ensuring smooth performance during peak times.
- Assume 100K daily active users and 500-1,000 events at any given time during peak periods.
- Storage required per user is 1 MB, so for 100K users, it totals 1 TB for user profiles.
- With 1K ticket purchases per second, the system would need approximately 100 TB for 1 million events, each with 100K tickets.
Clear estimations demonstrate planning and analytical skills crucial for system scalability and performance assessment.
3. Design Goals [5 mins]
Based on estimations and discussions, defining non-functional requirements for a Ticket Master system is important because they establish the criteria for performance, reliability, and user experience that are crucial for the system’s overall effectiveness.
- Strong Consistency: We need to prioritize consistency over availability to prevent double bookings.
- Availability: The system is read-heavy, as more users search for events than actually book tickets.
- Scalability: Scalability is crucial to properly handle event traffic
- Low Latency: Event searches should have minimal latency.
Specify latency/throughput targets and decide on consistency/availability levels based of estimations discussed for robust system design.
4. High-Level Design [5-8 mins]
To design a high-level system architecture for Ticket Master, we'll focus on the following aspects:
I. APIs for Read/Write Scenarios
Defining your APIs for Ticket Master is crucial because it establishes clear, standardized methods for interacting with your system, ensuring seamless integration between clients and the backend. Well-defined APIs enable efficient retrieval of event information, accurate search functionality, and reliable booking processes. This clarity enhances user experience by providing consistent and predictable responses, reduces the likelihood of errors, and facilitates easier maintenance and scaling of the system. By outlining precise endpoints and their functions, you streamline development, improve collaboration among teams, and support robust, scalable solutions for event management and ticketing.
Get Events
Endpoint | Parameters | Response |
GET /events |
{"event": [...array of events data...]} |
Get Specific Event Data
Endpoint | Parameters | Response |
GET /event/:eventID |
eventID |
{"eventID": [...details about the event...]} |
Search for Events
Endpoint | Parameters | Response |
GET search?term={filterText} |
filterText |
{"event": [...array of events data...]} |
Reserve Seat
Endpoint | Parameters | Response |
POST /booking/reserve |
eventID , venueID , seatID |
ticketID |
Confirm Booking
Endpoint | Parameters | Response |
POST /booking/confirm |
ticketID |
Confimation Status |
II. Database Schema
Defining your database schema is essential because it ensures a structured and organized approach to storing and retrieving data, which promotes data integrity and efficiency. For Ticket Master, choosing NoSQL over SQL is advantageous due to NoSQL's flexibility in handling unstructured or semi-structured data, which is common in event management systems where data types and structures can vary significantly. NoSQL databases offer horizontal scalability, allowing the system to handle large volumes of diverse and dynamic data seamlessly, and provide the ability to quickly adapt to changing requirements, which is crucial for managing event details and user interactions in a rapidly evolving environment.
Users Table
"userId": "UUID",
"username": "string",
"email": "string",
"passwordHash": "string",
"histroy": {list of events}
}
Event Table
"eventID": "UUID",
"name": "string",
"description": "string",
"venue": "venueID",
"performer": "performerID",
"status": "string",
"eventDate": "timestamp",
"ticketsSold": "number"
}
Venue Table
"venueID": "UUID",
"name": "string",
"location": "string",
"numberOfSeats": "number",
"bookings": "number",
"seatMap": {list of seats},
}
Performer Table
"performerID": "UUID",
"name": "string",
"description": "string",
"shows": {list of shows},
}
Ticket Table
"ticketID": "UUID",
"userID": "UUID",
"eventID": "UUID",
"seatID": "UUID",
"ticketPrice": "number",
"ticketStatus": {booked / not booked},
}
Ensure clear and concise communication of design choices and their implications to demonstrate deep understanding and critical thinking.
5. Deep Dive [10-12 mins]
Designing Ticket Master from users to main logic is crucial because it ensures that the system is built with a user-centric approach, focusing on delivering a seamless and intuitive experience. By starting with user requirements and interactions, you align the system's features and workflows with actual needs and expectations, which enhances usability and satisfaction. This user-focused design approach helps in identifying key functionalities and interactions early on, allowing you to create an efficient and responsive main logic that handles bookings, searches, and event management effectively. Ultimately, this method ensures that the system is not only robust and scalable but also tailored to provide a smooth, engaging experience for users.1. Users
End-users are the primary actors who interact with the ticketing platform. They use the system to search for events, book tickets, manage their user accounts, and receive notifications about their bookings or upcoming events. Users connect to the system over the internet, sending requests via a web or mobile interface. These requests are routed through various services to fulfill their needs.
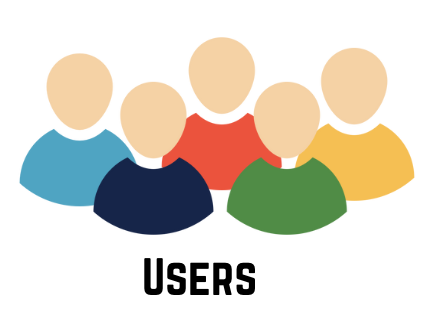
2. Load Balancer
Load Balancer is a critical component that distributes incoming user requests across multiple server instances. This ensures that no single server becomes a bottleneck, thereby optimizing resource utilization and enhancing the system's resilience to high traffic volumes. It manages the traffic flow to various microservices like the API Gateway, ensuring that each service receives requests in a balanced and efficient manner.
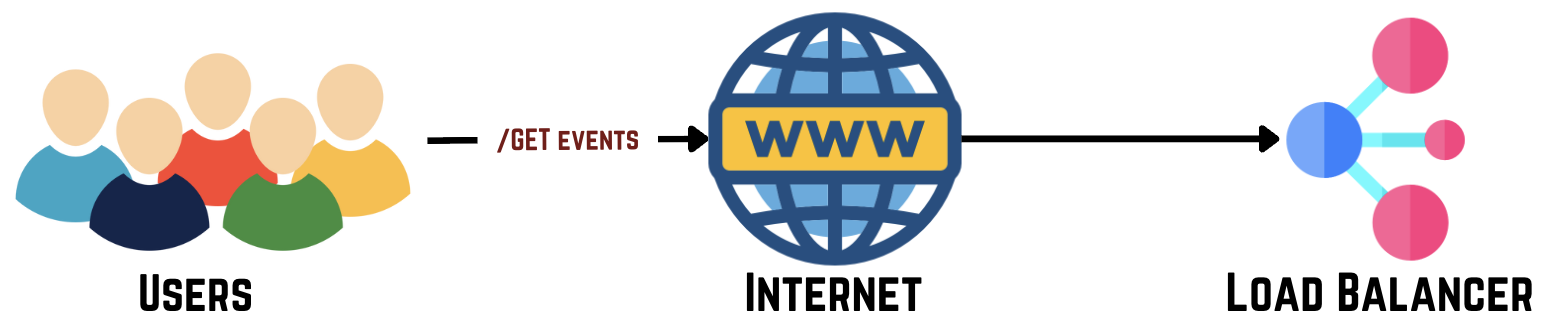
3. API Gateway
API Gateway acts as a centralized entry point for all user requests. It routes these requests to the appropriate backend microservices based on the type of request, such as searching for events, booking tickets, or managing user accounts. It interfaces with all microservices, ensuring smooth and secure communication between the client and the backend services.
- GET /events: Get a list of all upcoming events with details like name, date, location, and availability.
- GET /event/:eventID: Get detailed information about a specific event using its eventID.
- GET search?term={filterText}: Search for events based on a given search term.
- POST /booking/reserve: Reserve tickets for a specified event allowing users to initiate a reservation for tickets, holding the selected seats for a specified duration.
- POST /booking/confirm: Confirm the reservation and complete the booking process.

4. User Service
User Service manages all user-related functionalities, including user registration, authentication, and profile management. Interacts with the User Database to store and retrieve user information, such as account details, preferences, and transaction history. Handles requests related to user activities, such as logging in, updating profiles, and managing account settings.

5. User Database
User Database stores all user-related information, including account credentials, personal data, preferences, and transaction history. It is the primary data source for the User Service, ensuring that user data is securely stored and efficiently retrieved.

6. Event Service
Event Service is responsible for managing all event-related operations, including creating, updating, and retrieving event details. Interacts with the Event Database to maintain accurate and up-to-date information about events. It serves as the backbone for event data, ensuring that all event-related queries, updates, and searches are handled efficiently.
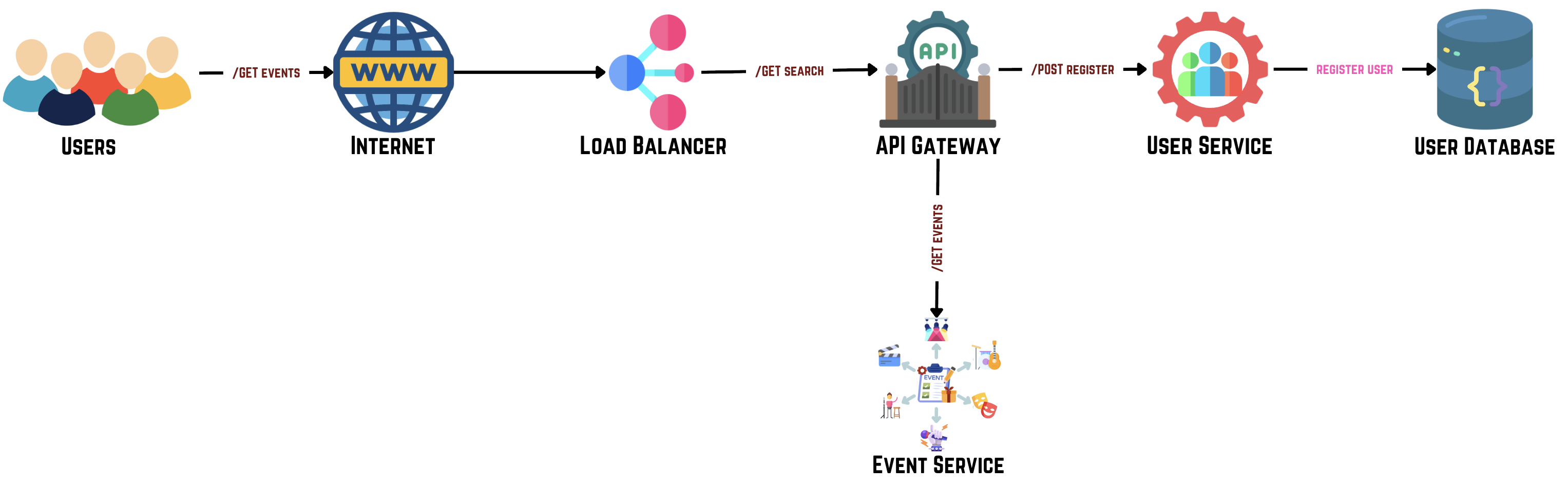
7. Event Database
Event Database is the primary repository for all event-related data, including event names, dates, venues, descriptions, and ticket types. It interacts with both the Search Service and Event Service to provide and manage event information as required by the system.
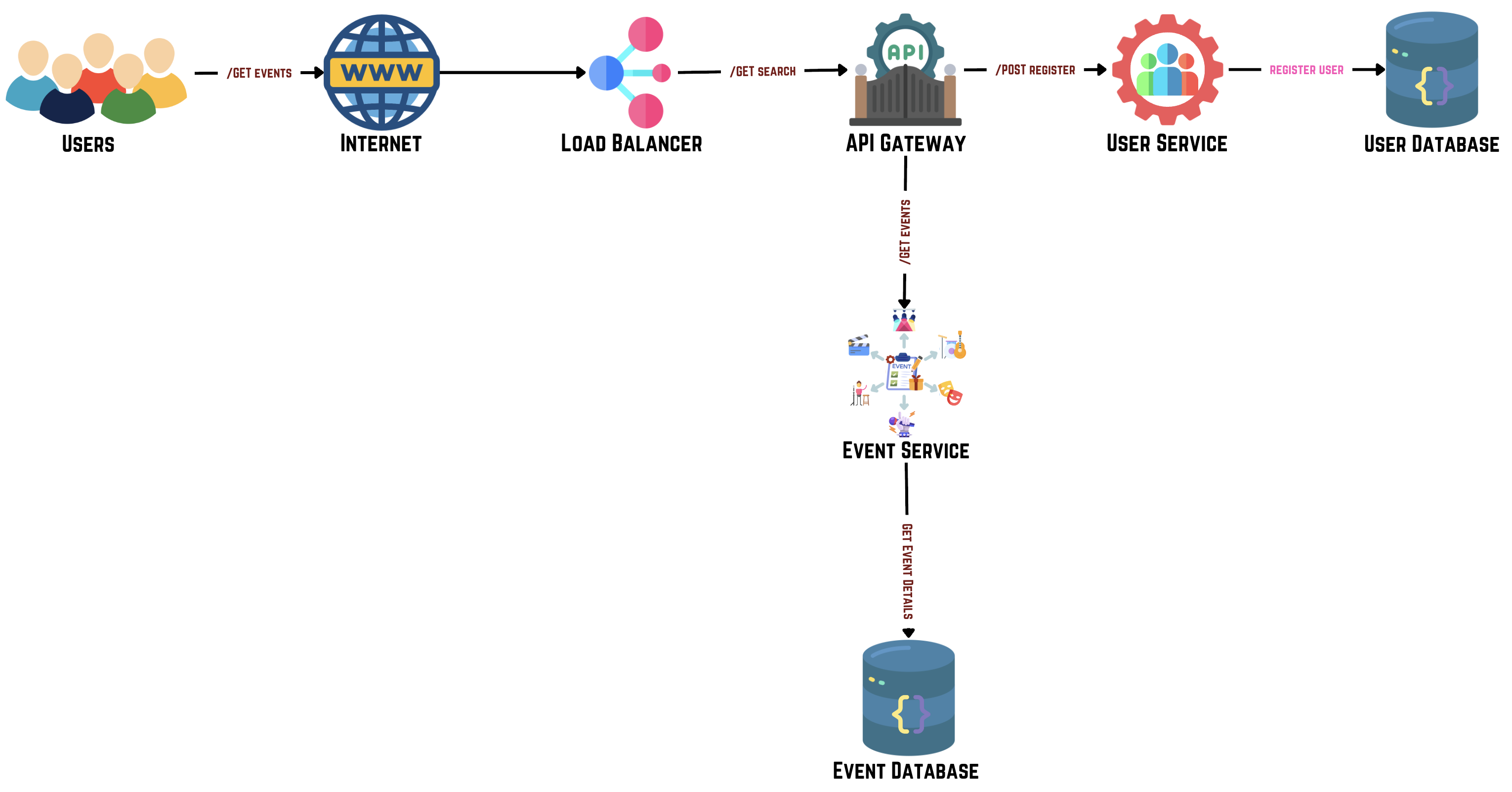
8. Performer Database
This database stores detailed information about performers, such as artists, bands, and other entertainment entities. It accesses this database to fetch performer details during user searches.
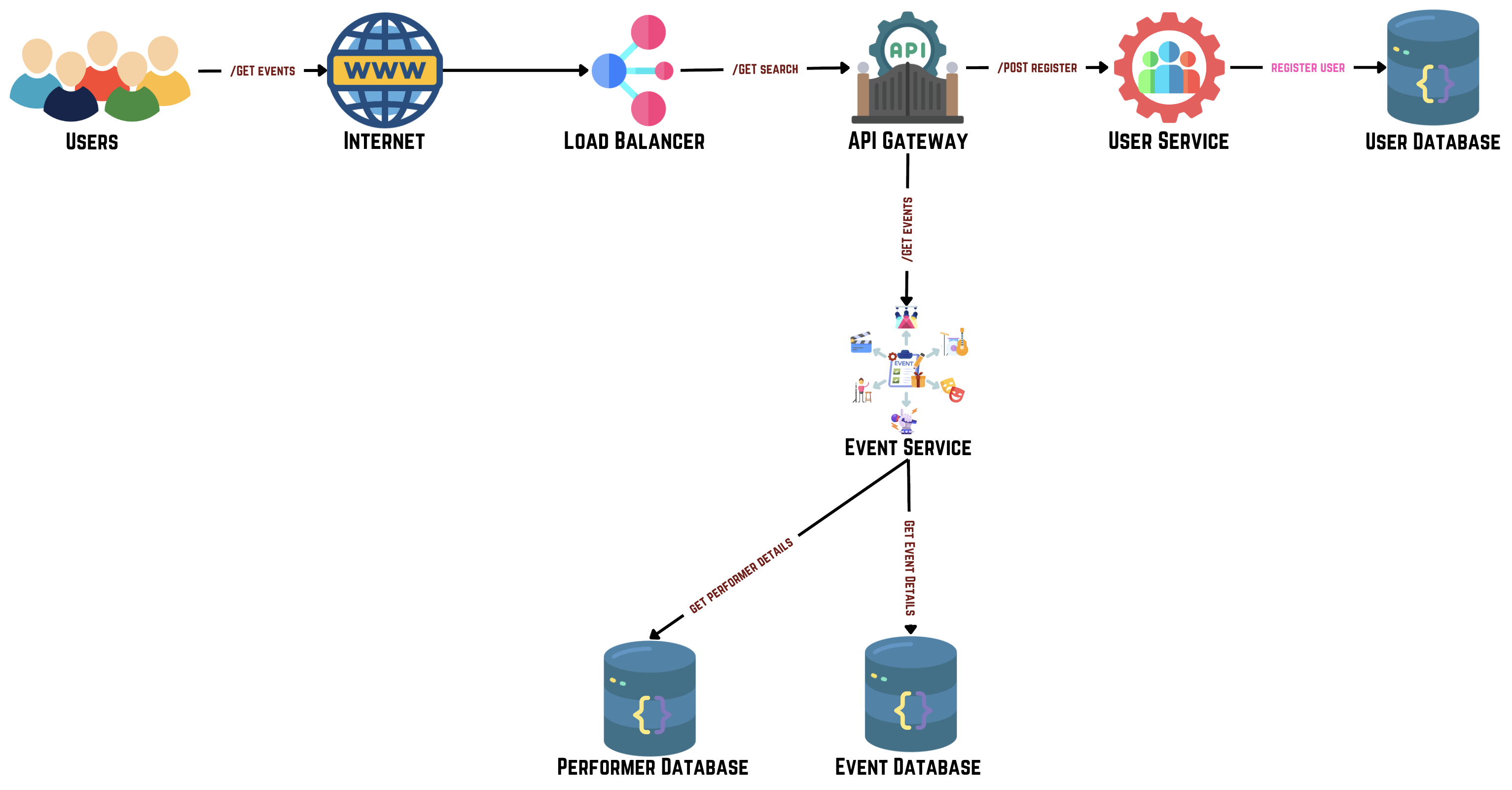
9. Venue Database
Stores information about venues where events take place, including details like seating layouts, capacities, and locations. This database is accessed by the Event Service and Search Service to manage and retrieve venue-specific data, ensuring that users get accurate venue information when booking tickets or searching for events.
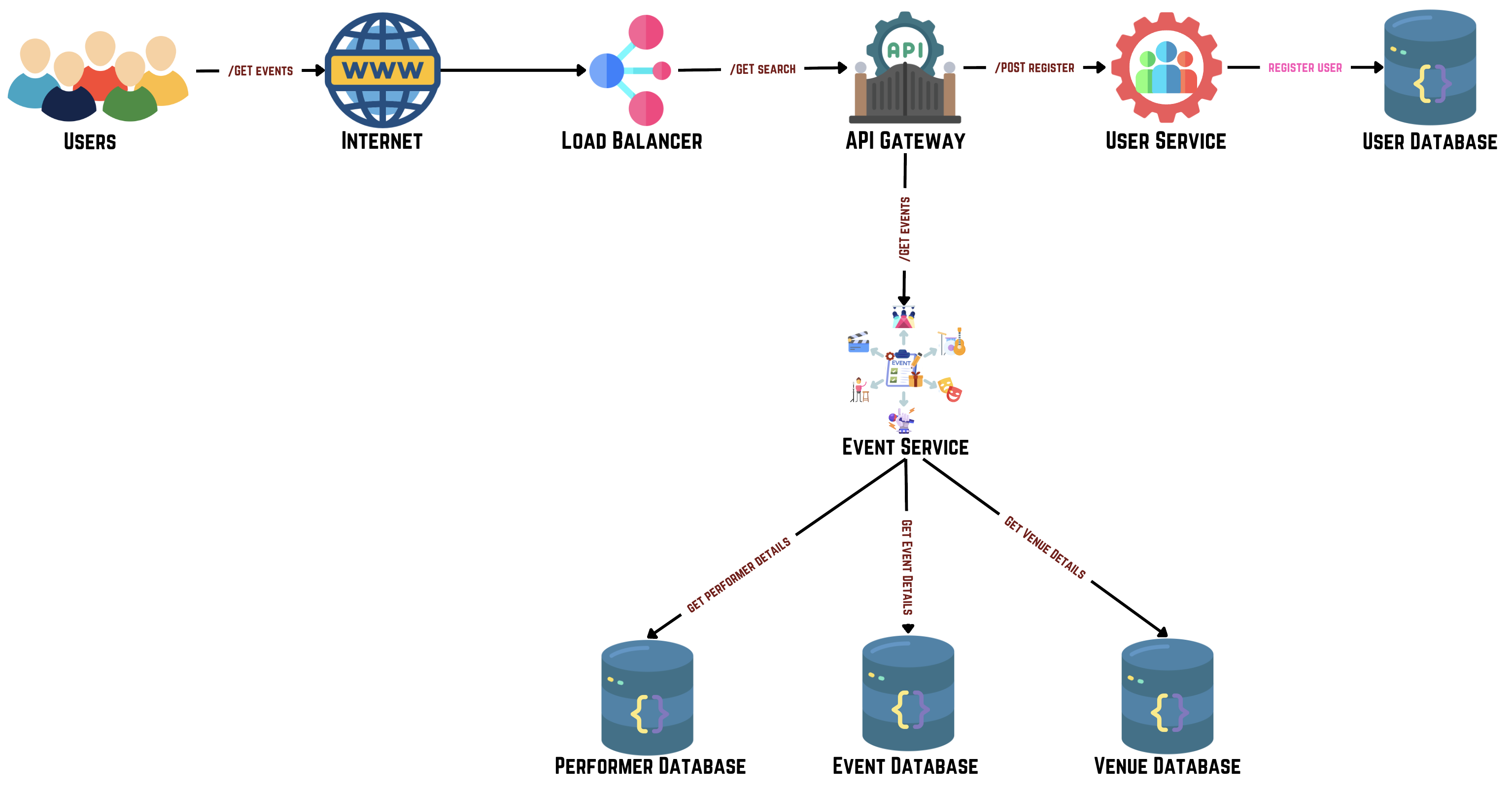
10. Search Service
Search Service handles all search-related queries from users, such as searching for events, performers, or venues. It processes search queries and fetches results from the corresponding databases, enhancing the user experience with efficient data retrieval.
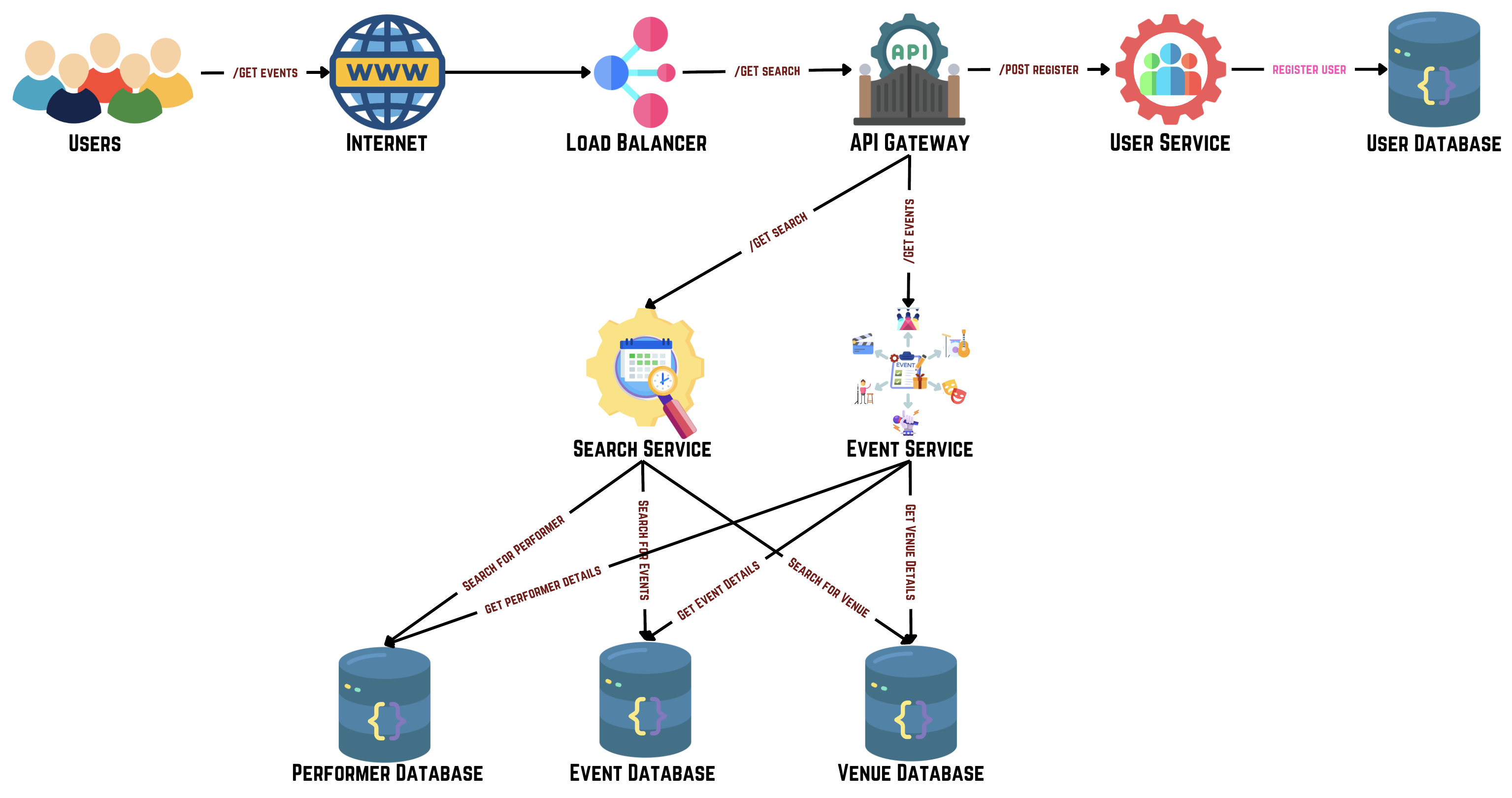
11. Booking Service
Booking Service manages the entire ticket reservation and purchasing process, including seat selection, order management, and payment processing. Interacts with the Ticket Database to manage ticket inventory, including tracking available seats, reservations, and sales. Works closely with the Payment Service to ensure secure and successful transactions before confirming a booking. It is a crucial component for managing user transactions, ensuring that ticket bookings are processed smoothly and securely.
Key functionalities include:- Seat Reservation: Communicates with the Distributed Ticket Lock to temporarily lock seats during the booking process, ensuring that seats are not double-booked.
- Order Management: Handles the full lifecycle of ticket orders, from initial reservation to final purchase.
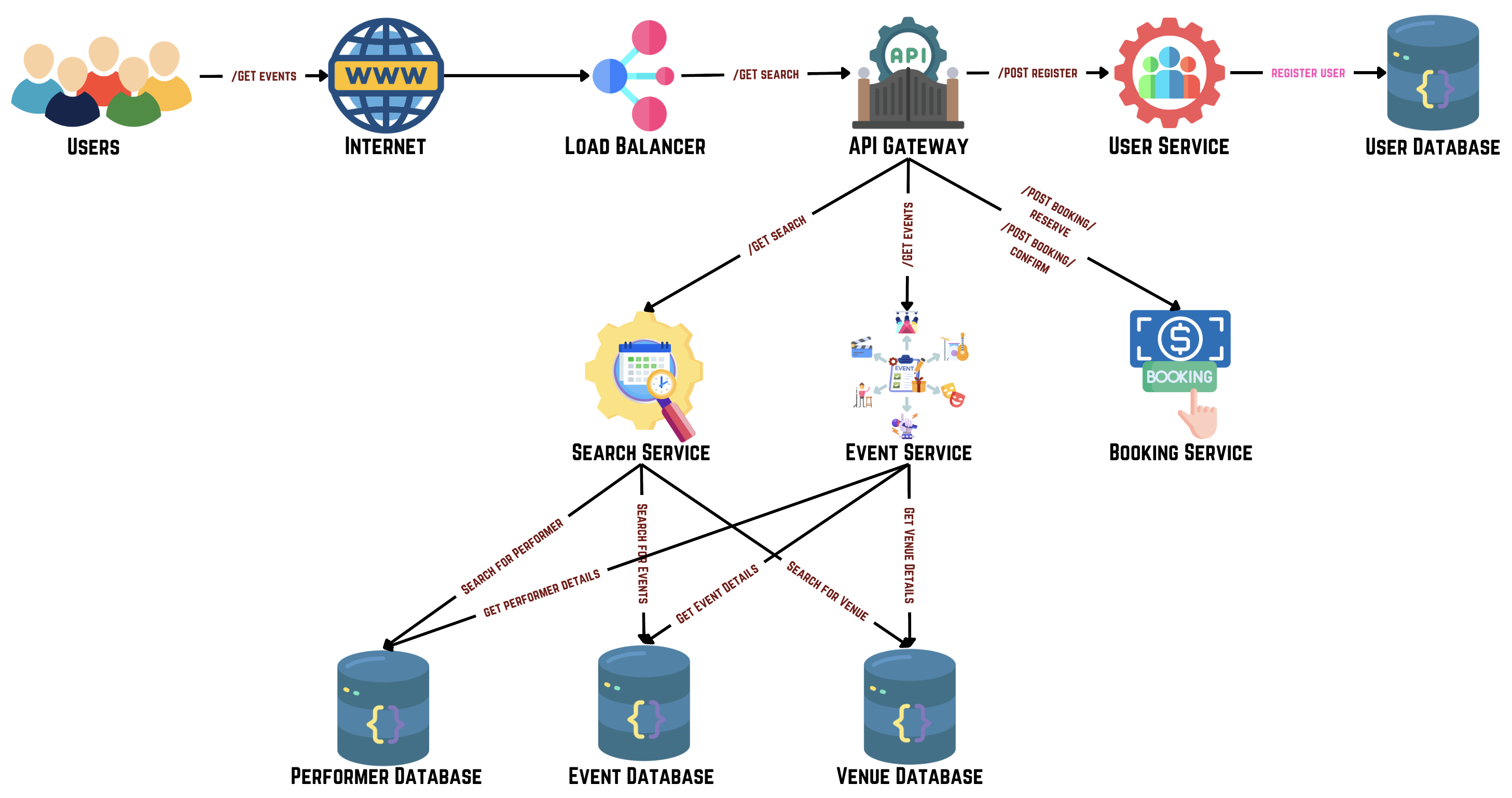
12. Distributed Ticket Lock
Distributed Ticket Lock is essential for maintaining the integrity of the ticketing system, preventing double bookings by temporarily locking tickets during the reservation process. Booking Service communicates with the Distributed Ticket Lock to lock and unlock seats during the reservation process. This mechanism helps maintain accurate ticket inventory and prevents overselling.
Distributed Ticket Lock is especially critical in scenarios where there is high concurrency, such as during the sale of tickets for popular events. It ensures that once a user initiates a booking process, the selected seats are locked for a short duration (e.g., 10 minutes), allowing the user to complete the purchase without interference from other users.
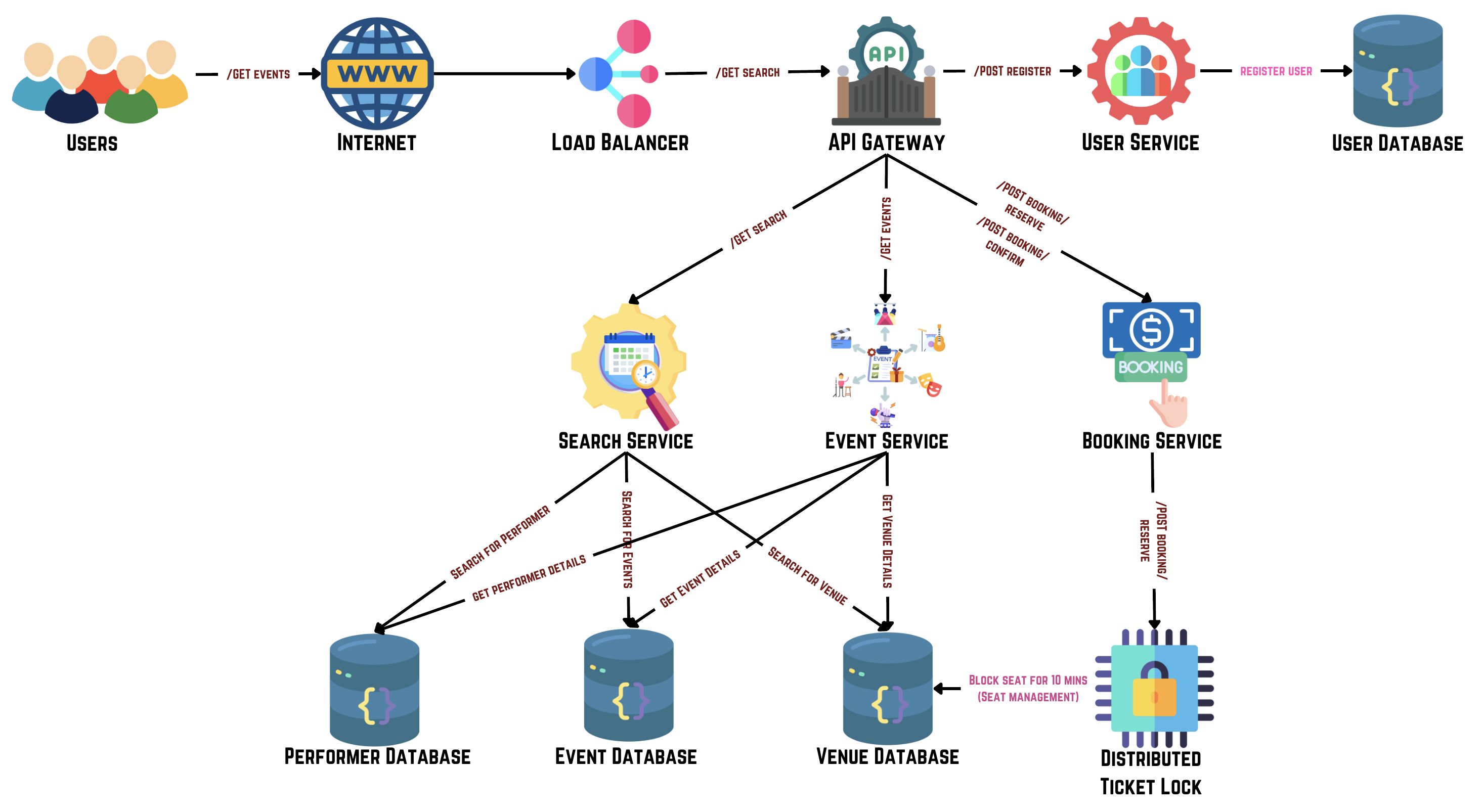
13. Payment Service
Payment Service handles the financial transactions associated with ticket purchases. It integrates with external payment gateways to process payments securely, manage refunds, and handle other financial aspects. Once a user confirms a booking, the Booking Service hands off the transaction to the Payment Service, which processes the payment. Upon successful payment, the Payment Service notifies the Booking Service to finalize the ticket purchase. It also interacts with the Notification Service to inform users about payment confirmation and any issues.
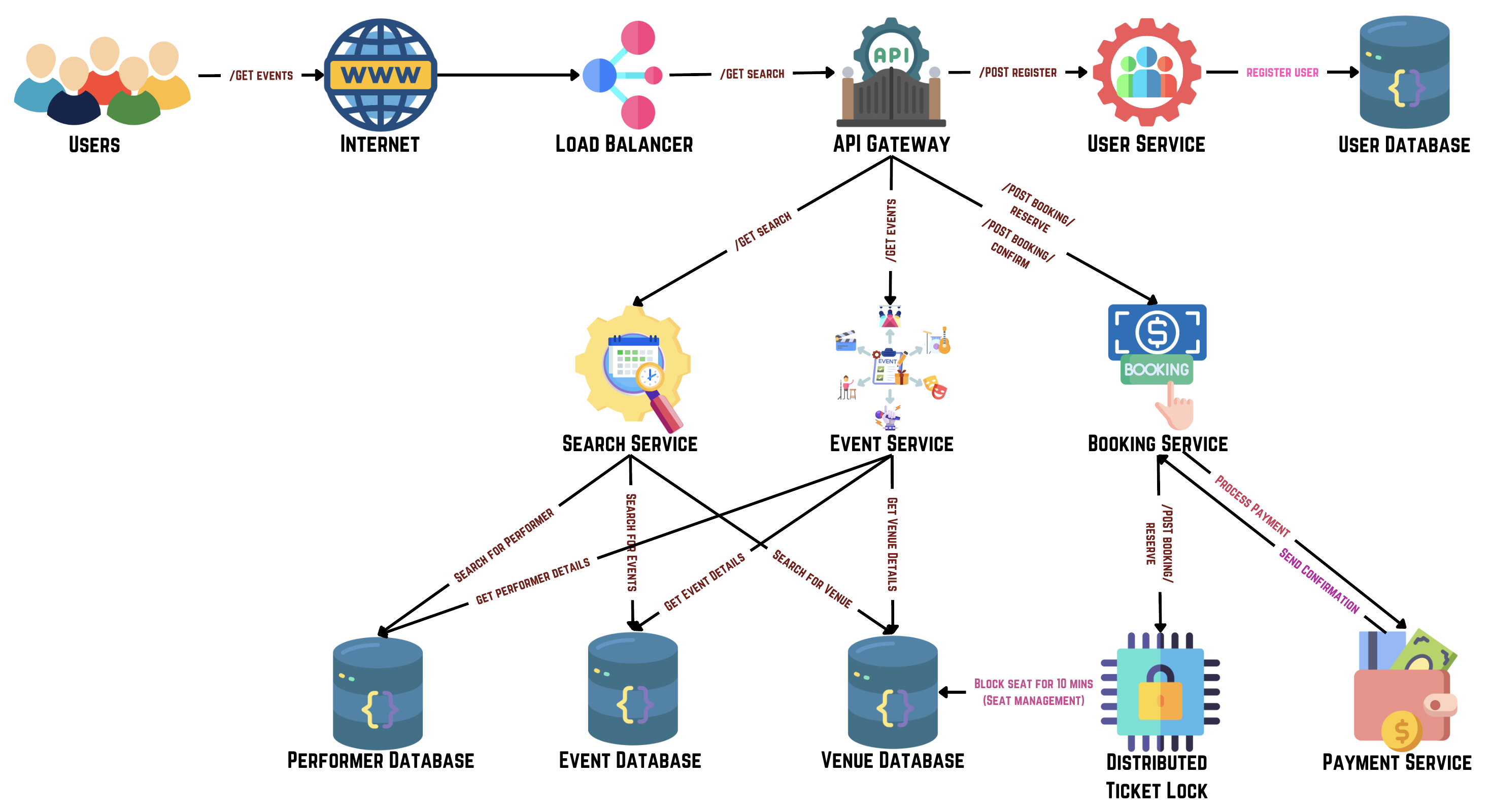
14. Ticket Database
Ticket Database is responsible for storing and managing all ticket-related data, including available tickets, reserved tickets, and sales records. It works in tandem with the Booking Service to update ticket availability in real time as users make reservations and complete purchases. The database ensures that the ticketing system accurately reflects the current inventory at all times.
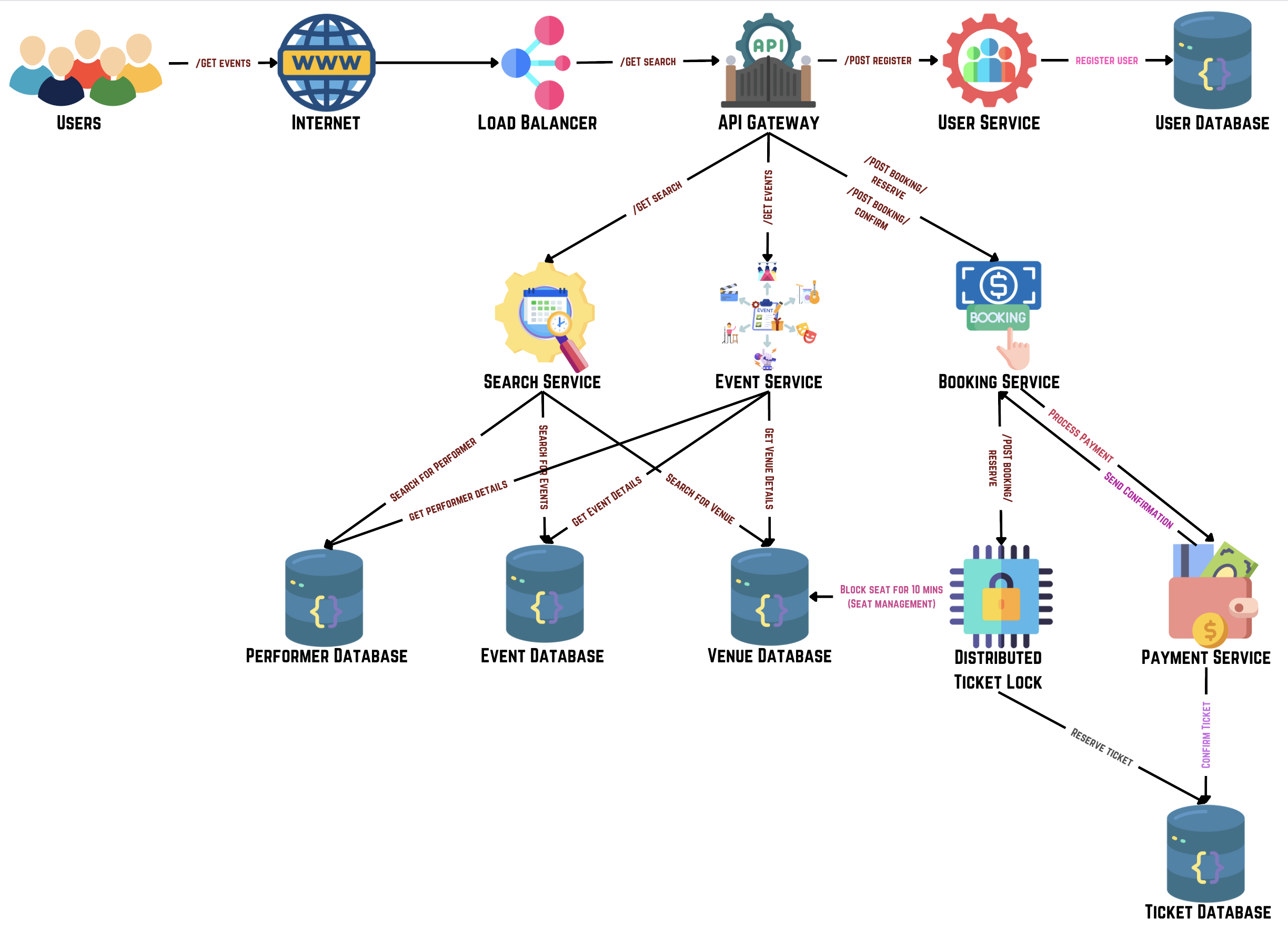
15. Notification Service
Notification Service is responsible for sending out various notifications to users, including booking confirmations, event reminders, and promotional messages. This service works with multiple components such as the Booking Service and User Service to trigger notifications based on specific events, such as a successful booking or an upcoming event. Notifications can be sent via email, SMS, or in-app alerts, ensuring that users are kept informed throughout their interaction with the platform.
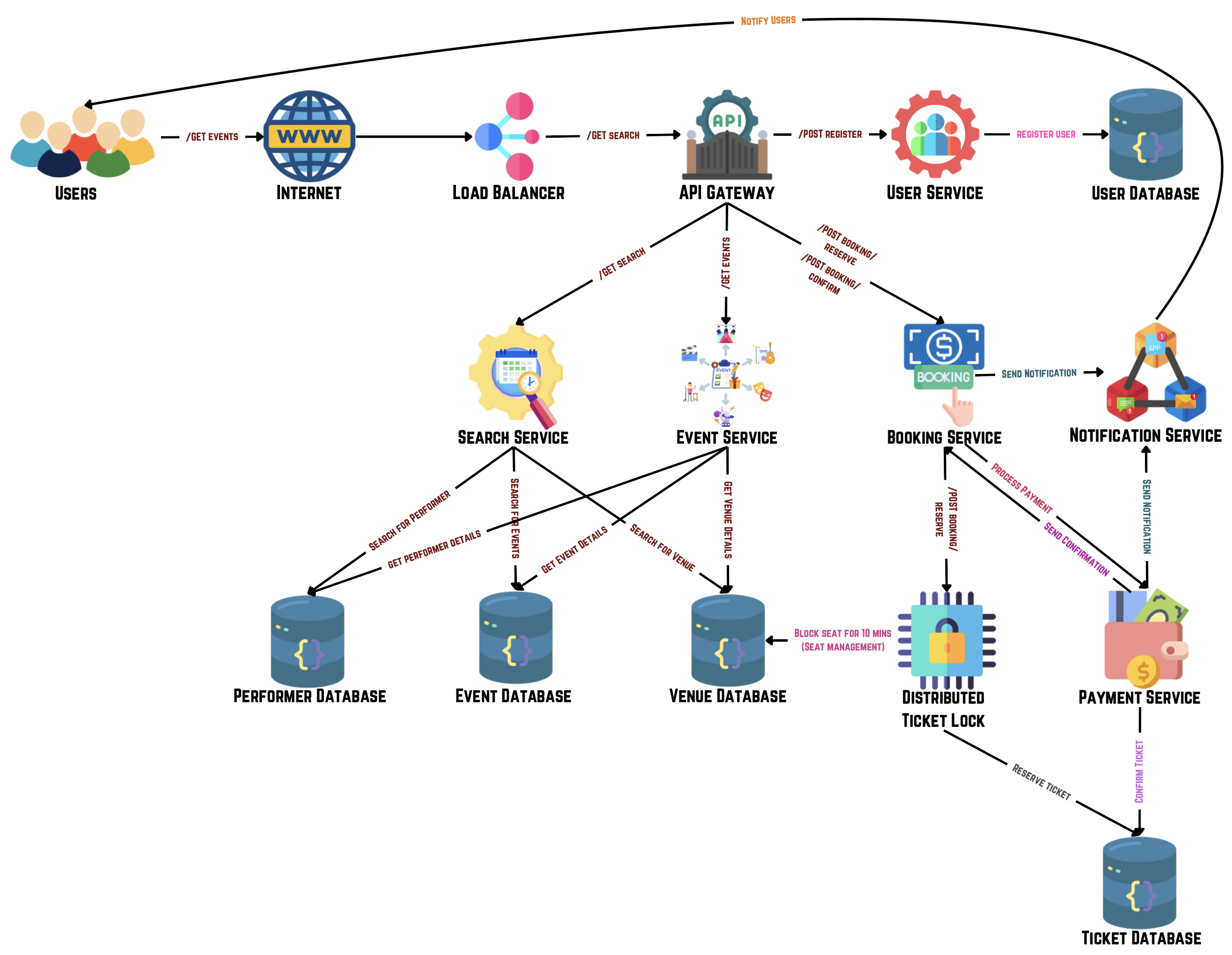
6. Futher Optimizations [2 - 5 mins]
1. Search Optimization using ElasticSearch
ElasticSearch is a search-optimized database that enhances the search functionality across the platform. It provides fast and efficient search results for queries related to events, performers, and venues. Search Service leverages ElasticSearch to quickly retrieve relevant information from the Performer, Event, and Venue databases. ElasticSearch indexes this data to make searches more responsive and scalable, especially when dealing with large datasets.
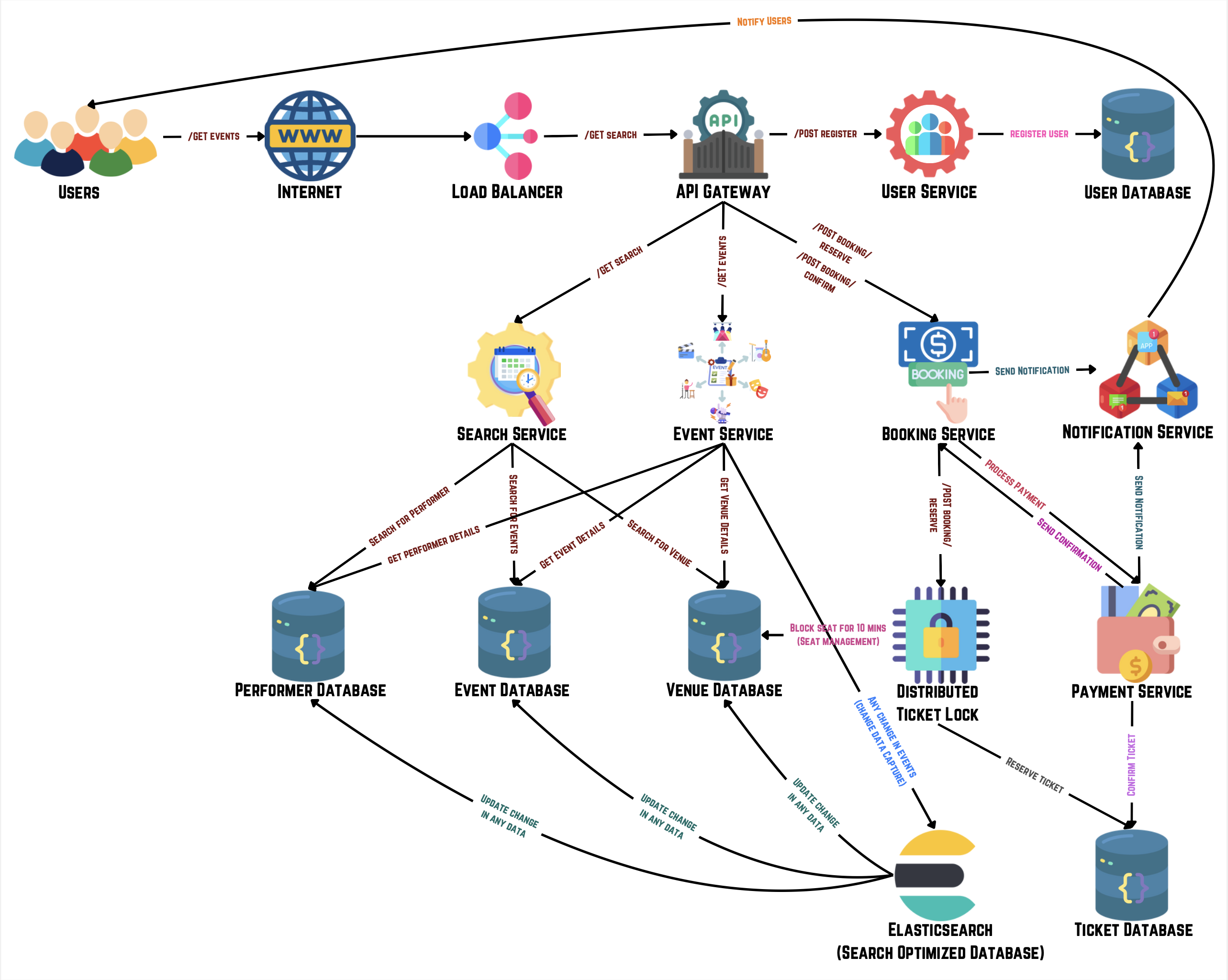
2. CDN (Content Delivery Network)
CDN (Content Delivery Network) is used to enhance performance and reliability by distributing static assets, such as images, CSS, and JavaScript files, across a network of geographically dispersed servers. This ensures faster load times and reduced latency for users accessing the site from various locations. Additionally, the CDN can cache and deliver frequently accessed event data, ticketing information, and search results, decreasing the load on the origin servers and improving response times. By offloading traffic to the CDN, Ticket Master can handle high volumes of concurrent users and traffic spikes more efficiently, providing a smoother and more responsive booking experience.
High Level Architecture of Ticket Master after Optimizations
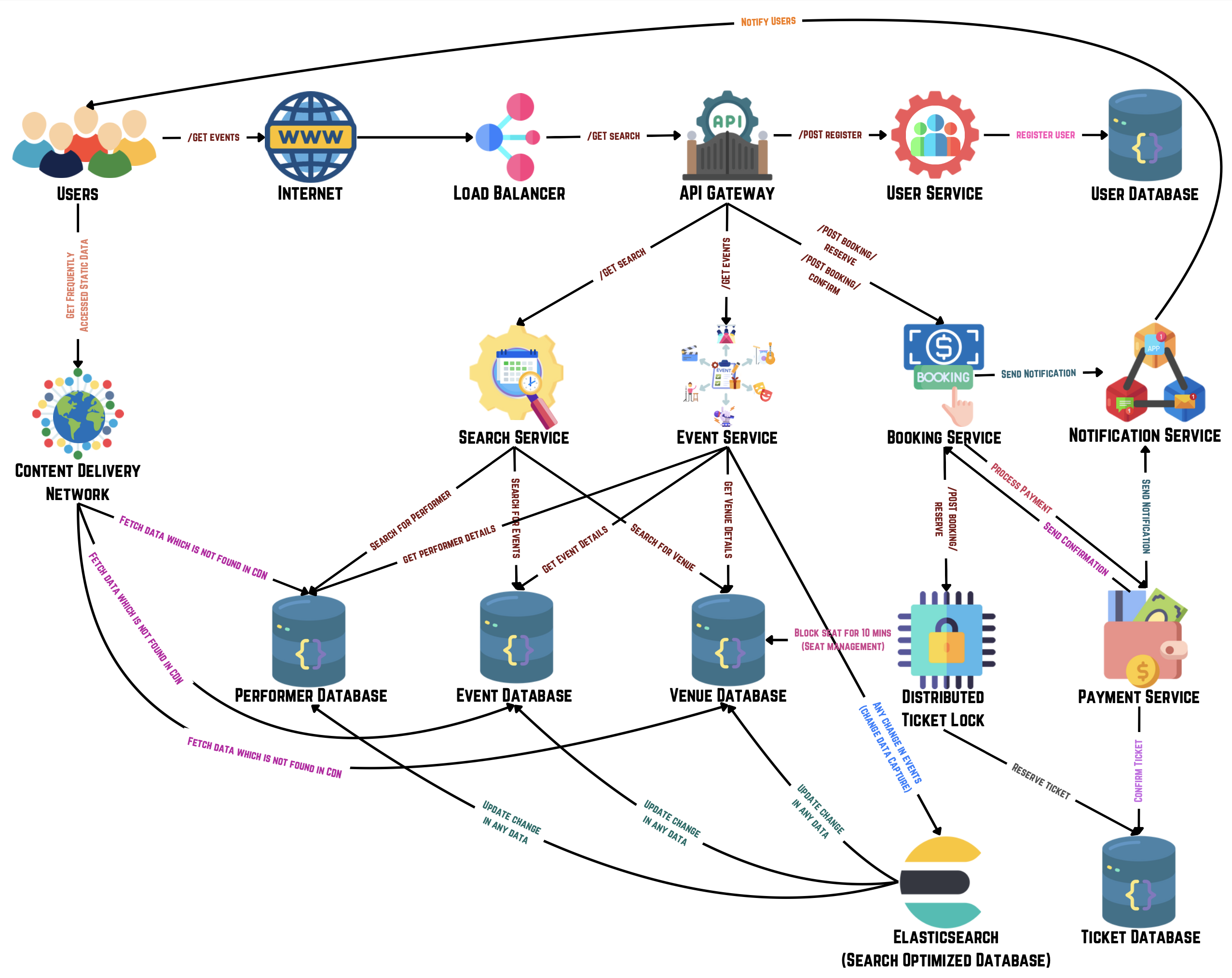
7. Data Flow [5-8 mins]
It's essential to explain the end-to-end flow of the design, ensuring clarity on how data moves through the system. Based on estimations and discussions, the non-functional requirements for the system include addressing critical aspects such as performance under high user loads, robust data security measures, and scalable infrastructure to accommodate rapid growth.
View Available Events:
- Users view all the available events.
- The request is sent to the API Gateway.
- The API Gateway routes the request to the Event Service.
- The Event Service retrieves the event list from the Events Database.
- The event details, including name, date, location, and availability, are presented to the user.
View Specific Event Details:
- Users select an event to see more detailed information.
- The request is sent to the API Gateway.
- The API Gateway routes the request to the Event Service.
- The Event Service retrieves detailed information for the selected event from the Events Database.
- The detailed event information is presented to the user.
Search for Events:
- Users search for events using a specific keyword or term.
- The request is sent to the API Gateway.
- The API Gateway routes the request to the Event Service.
- The Event Service searches the Events Database for matching events based on the filter text.
- The search results are presented to the user.
Reserve Tickets:
- Users initiate a reservation for tickets to a selected event.
- The request is sent to the API Gateway.
- The API Gateway receives the reservation request and routes it to the Booking Service.
- The Booking Service interacts with the Distributed Ticket Lock system to temporarily block seats in the Ticket Database for 10 minutes.
- Reservation details are stored, and the seats are temporarily held.
Booking Confirmation:
- Users confirm their reservation by submitting payment details.
- The API Gateway routes this request to the Payment Service.
- The Payment Service processes the payment and confirms the reservation in the Ticket Database.
- The Booking Service finalizes the booking and updates the seat status from held to reserved.
- Once the booking is confirmed, the Notification Service is triggered to send confirmation notifications including booking ID and ticket information is sent to the user.
Don't forget to explain the end to end flow of your design!
This architecture is designed to be scalable, resilient, and efficient, ensuring that the platform can handle a high volume of user interactions and data processing with minimal latency and high availability.