Pastebin System Design
Never dive directly into the design phase; it may raise red flags during the interview!
Interviewer: Can you design a pastebin-like application for us?
1. Feature Expectations [5 mins]
You: Could you outline our main use cases and key requirements?
Interviewer: We need users to securely store and share text snippets. How do you propose handling them?
You: To keep text snippets secure, we'll use strong user authentication, encrypt data both while it's moving and when it's stored, control who can access each snippet, keep detailed logs of access, and use secure methods for sharing snippets through the app. These steps make sure user data is safe from unauthorized access and keeps the sharing of snippets controlled and managed.
You: Should pastes have unique URLs, and how long should they remain accessible?
Interviewer: Unique URLs and customizable visibility are crucial.
You: Thank you for clarifying the requirements. Based on our discussion, here are the identified functional requirements:
Functional Requirements
- Paste Creation
- Paste Management
- Sharing and Access Control
Pastebin serves a diverse user base, including developers, programmers, students, educators, and anyone needing a simple platform to share text-based information online. Scalability planning considers the platform's millions of active users worldwide, ensuring it can handle concurrent accesses and uploads efficiently.
Users typically engage with Pastebin to share snippets of code, technical notes, and other textual content. Usage patterns range from occasional use for individual sharing to intensive use by power users involved in collaborative coding or sharing technical documentation.
Limit the number of features discussed to one or two, as covering more can be time-consuming and may detract from explaining the most critical aspects of the design.
2. Estimations [5 mins]
Estimations for designing a pastebin-like application involve projecting user base growth, estimating data storage needs, and predicting system load based on anticipated user activity. These estimates guide infrastructure planning and ensure the application can handle current and future demands effectively.
- Assuming 1 million active users per day
- Average of 2 pastes per user per day
- 1 million active users * 2 pastes per day = 2 million pastes per day
- Assuming each paste is 1MB in size i.e. storage for 1 paste = 1MB * 2 million pastes = 2TB per day
- Peak Transactions Per Minute (TPM) estimated at 1,000 for handling paste uploads on Pastebin.
Clear estimations demonstrate planning and analytical skills crucial for system scalability and performance assessment.
3. Design Goals [5 mins]
To meet these estimations, designing the pastebin application requires focusing on non-functional requirements like scalability for handling more users, strong security to protect data, and efficient performance to manage large amounts of text smoothly. These goals ensure the application works reliably, securely, and quickly for users.- Performance
- Reliability
- Security
- Scalability
Specify latency/throughput targets and decide on consistency/availability levels based of estimations discussed for robust system design.
4. High-Level Design [5-8 mins]
Let's design a high-level system architecture for a platform similar to Pastebin, focusing on APIs for read/write scenarios, database schema, core algorithms, and overall architecture for handling operations.
I. APIs for Read/Write Scenarios
Designing APIs is crucial in Pastebin's system design because they define how different parts of the system communicate. Clear and efficient APIs for reading and writing data ensure smooth interaction between the frontend and backend, facilitate easy data handling, support future scalability, and enhance code reusability and manageability.
Get Paste
Endpoint | Parameters | Response |
GET /pastes/{paste_id} |
paste_id |
Paste data (paste_id, user_id, content, timestamp, visibility) |
Create Paste
Endpoint | Parameters | Response |
POST /pastes |
user_id , content , visibility |
Created paste data (paste_id, user_id, content, timestamp) |
II. Database Schema
Establishing a well-structured database schema is essential for Pastebin as it defines how data is organized and accessed. A carefully designed schema ensures efficient storage and retrieval of text snippets, supports optimal query performance, and maintains data integrity across the application.
Users Table
"user_id": "UUID",
"username": "string",
"email": "string",
"password_hash": "string",
"created_at": "timestamp"
}
Keys Table
"key_id": "UUID",
"hash_key": "string",
"created_at": "timestamp"
}
Pastes Table
"paste_id": "UUID",
"key_id": "UUID",
"content": "string",
"visibility": "string",
"created_at": "timestamp"
}
Ensure clear and concise communication of design choices and their implications to demonstrate deep understanding and critical thinking.
5. Deep Dive [10-12 mins]
Let's carefully plan the system architecture for Pastebin to ensure it meets our requirements effectively. Taking a step-by-step approach helps us prevent errors and guarantees the system's reliability, supporting seamless growth and scalability over time.
1. Users
Users interact with the paste-bin service by creating new pastes or retrieving existing ones via URLs. This simple interface belies the complex system behind it, designed to handle potentially millions of interactions efficiently and securely.
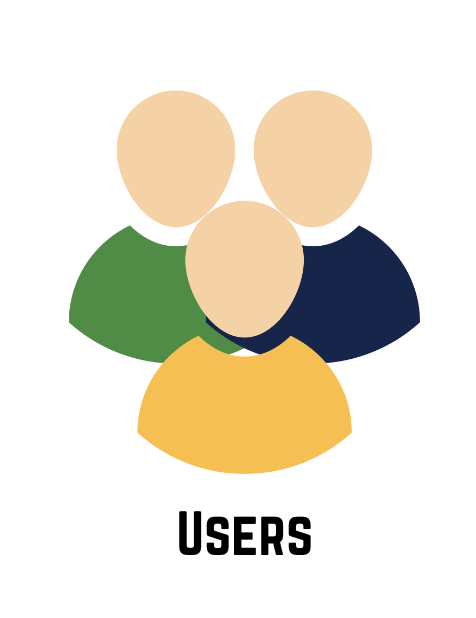
2. Load Balancer
The Load Balancer serves as the front door to the service, intelligently distributing incoming requests across multiple app servers. This crucial component ensures no single server becomes overwhelmed, maintaining high availability and responsiveness even under heavy traffic conditions.
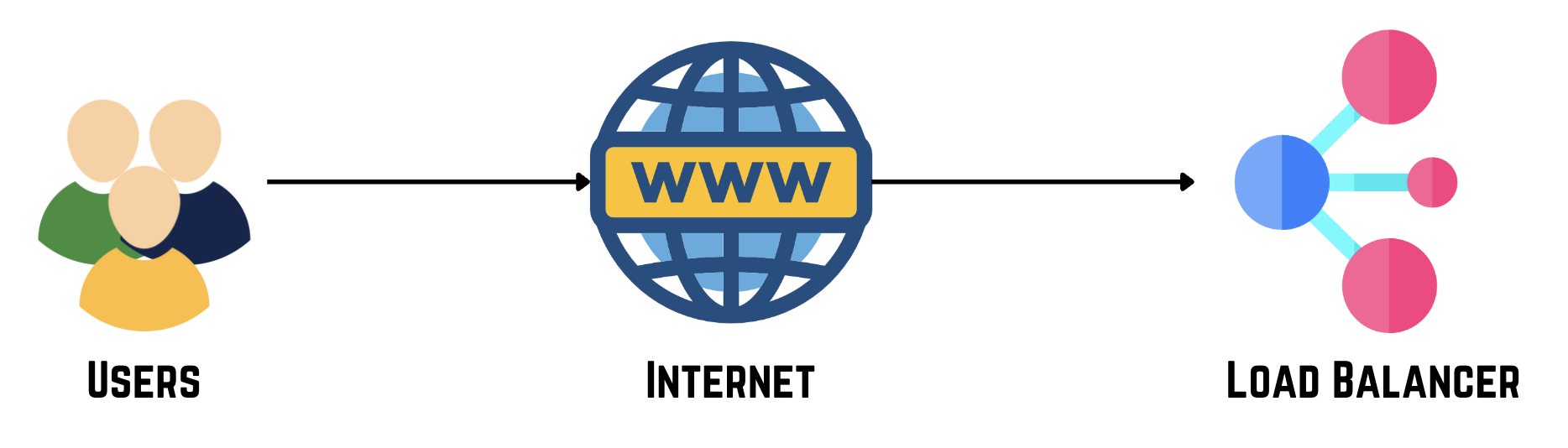
3. API Gateway
It acts as a single point of entry for all API calls, consolidating various microservices or backend services behind a unified API interface. It performs tasks such as request routing, protocol translation, authentication, authorization, and traffic management (like rate limiting).
- /POST Paste: Directs the request to post a new paste.
- /GET Paste: Routes the request to retrieve pastes for the user.
- /PUT Paste: Updates an existing paste.
- /DELETE Paste: Deletes a specified paste.
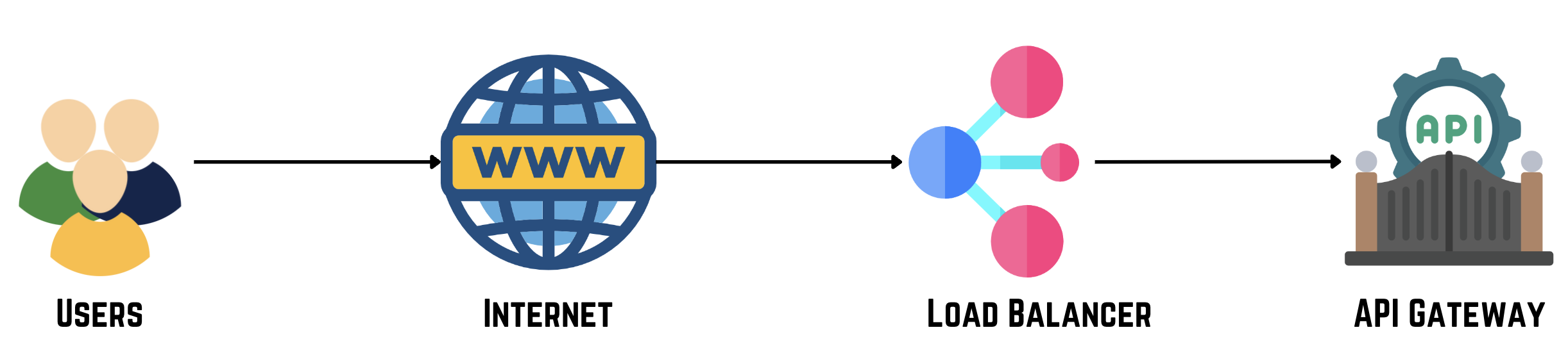
4. User Service
The user service manages user-related functionalities such as registration, authentication, profile management, and user-specific data access within an application or system.

5. User Database
The User Database stores essential information about registered users, likely including usernames, email addresses, and potentially encrypted passwords. This NoSQL database enables user management features and personalized experiences for registered users.
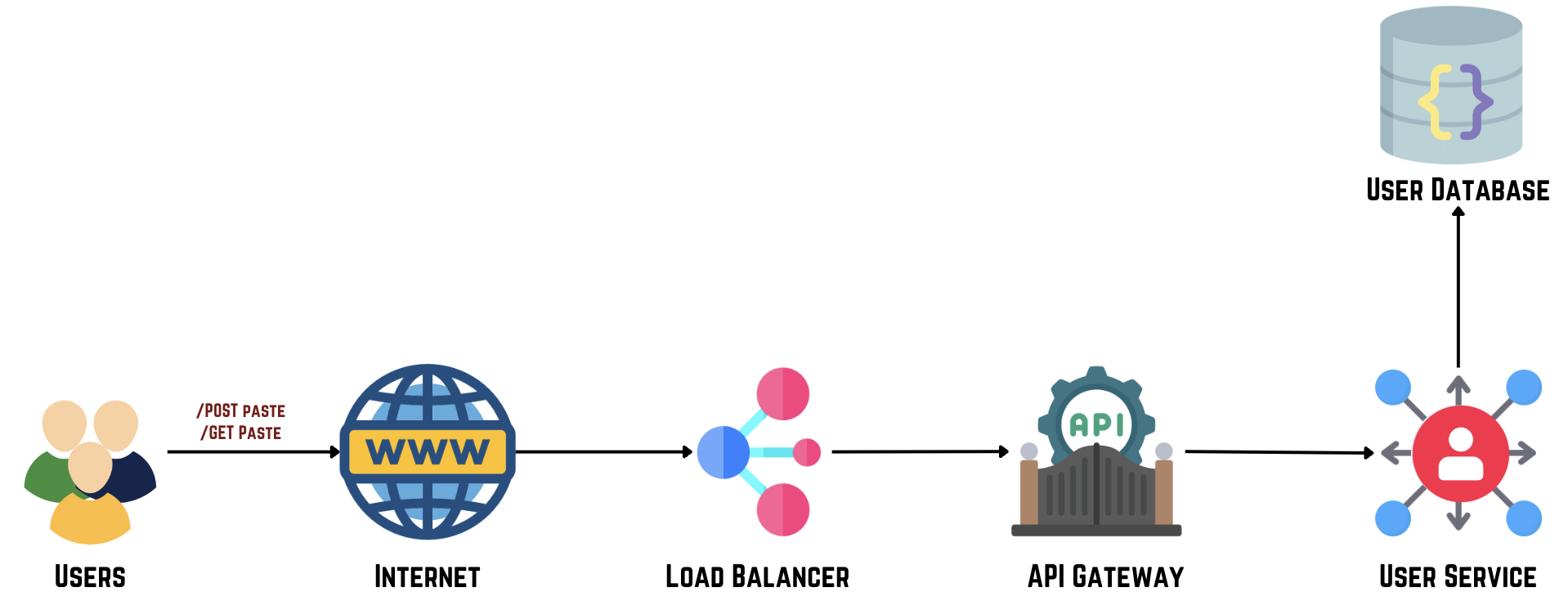
6. Paste Service
The paste service manages the creation, retrieval, update, and deletion of text or code snippets, including uploading content to a content storage system and updating the paste database accordingly.
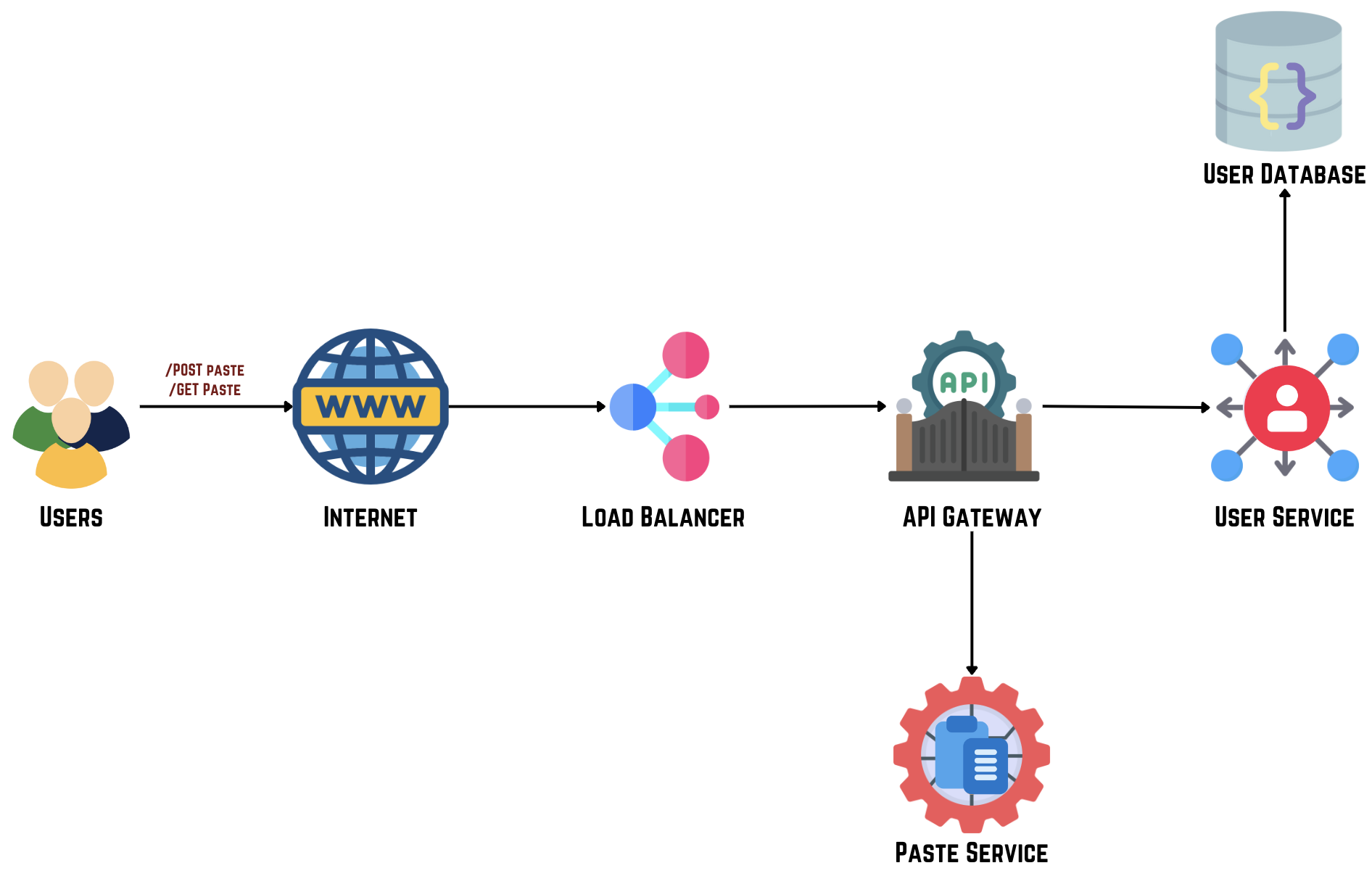
7. Content Storage
Content Storage contains the actual text content of the pastes. By separating content from metadata, this component allows for efficient storage and retrieval of potentially large volumes of text data.
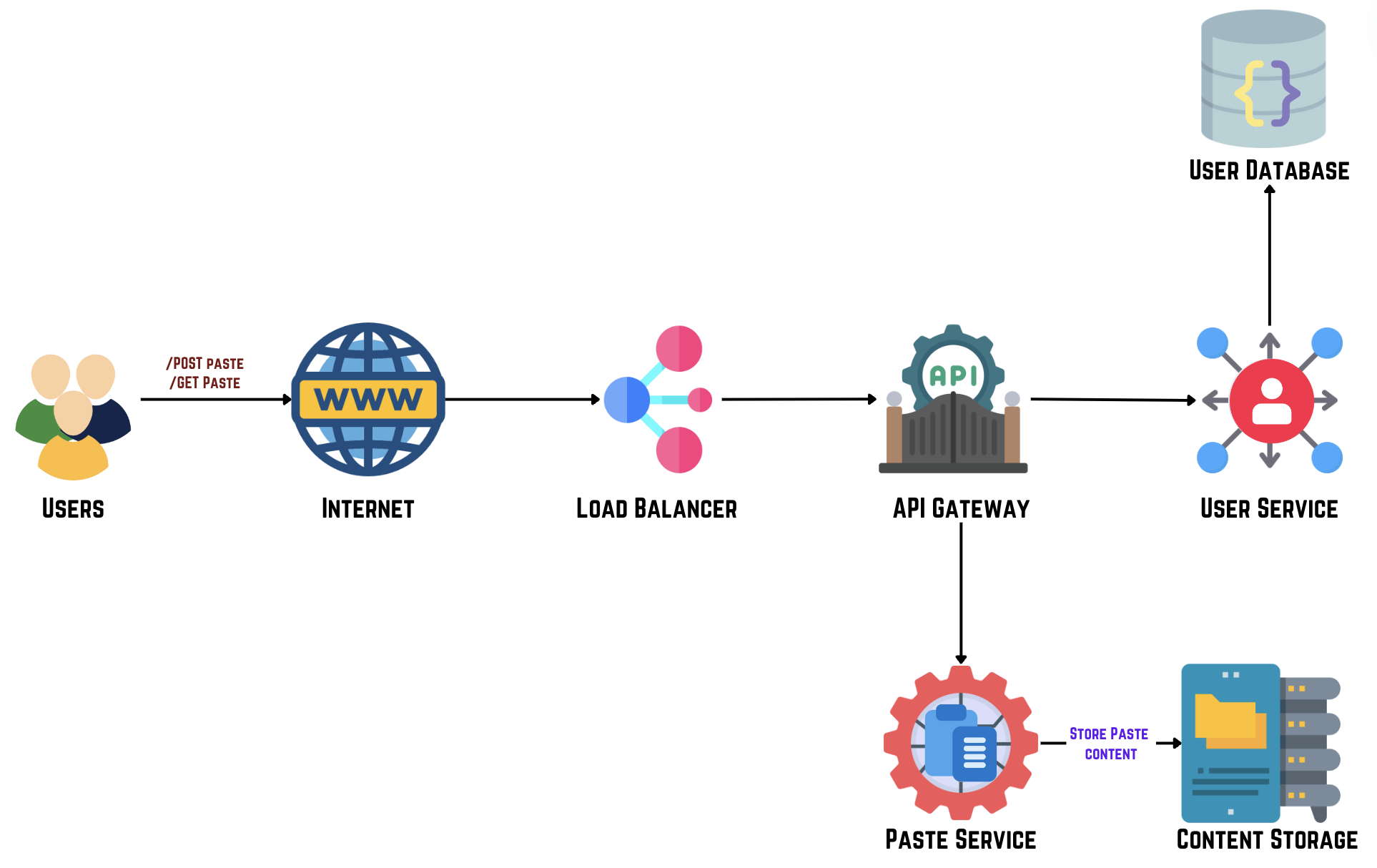
8. Key Generation Service
The Key Generation Service creates unique identifiers for each paste, ensuring that every piece of content has a distinct, easily shareable URL. This service might employ various techniques like hashing or UUID generation to create these identifiers efficiently and collision-free.
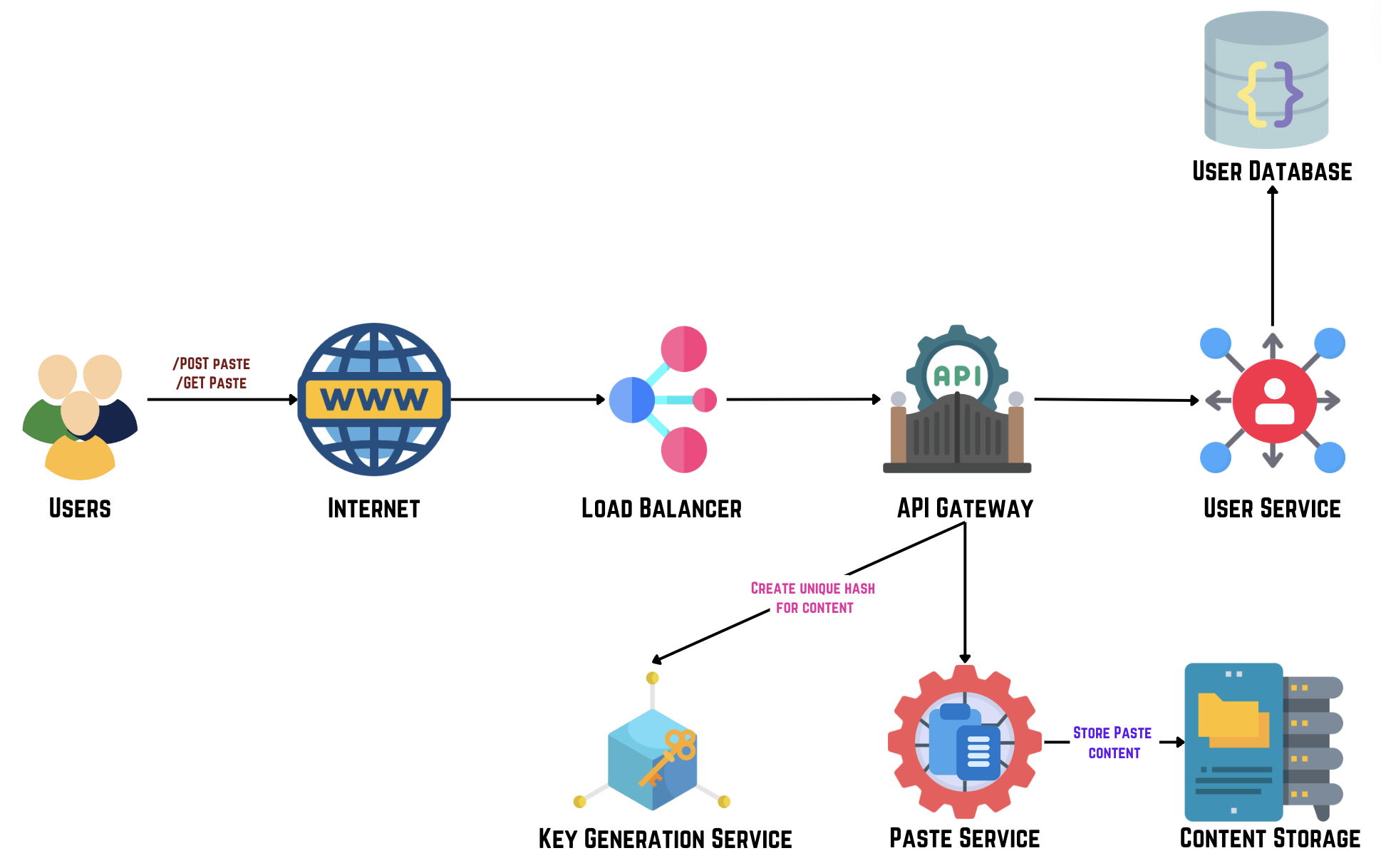
9. Key Database
The Key Database maintains crucial metadata about each paste, including custom URLs, content locations, and expiration dates. This separation of concerns allows for quick lookups and efficient management of paste lifecycle without needing to access the full content.
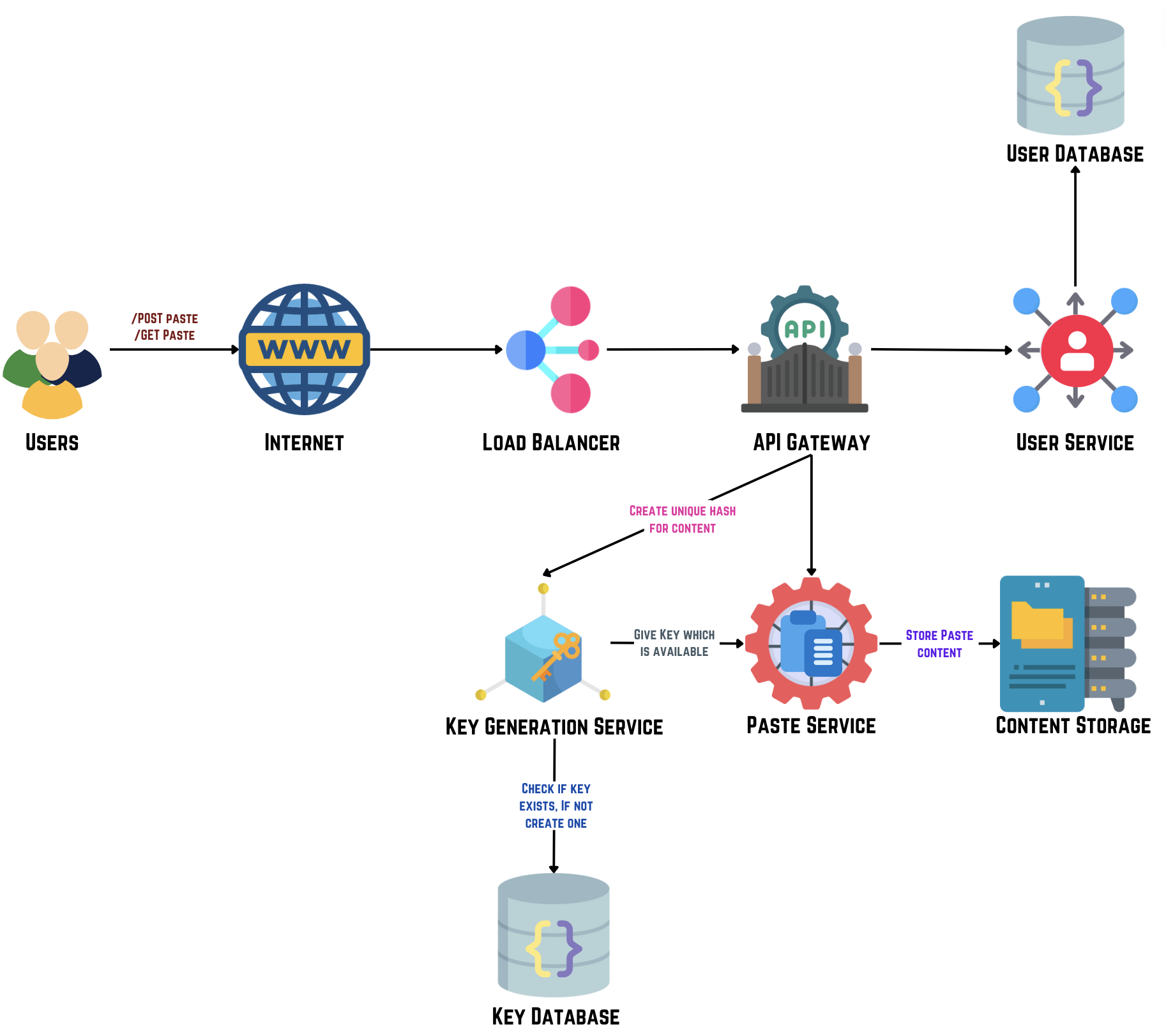
10. Paste Database
Paste Database stores structured data related to pastes, potentially serving as an index for the content storage or holding additional metadata. This component enables efficient querying and management of paste-related information.
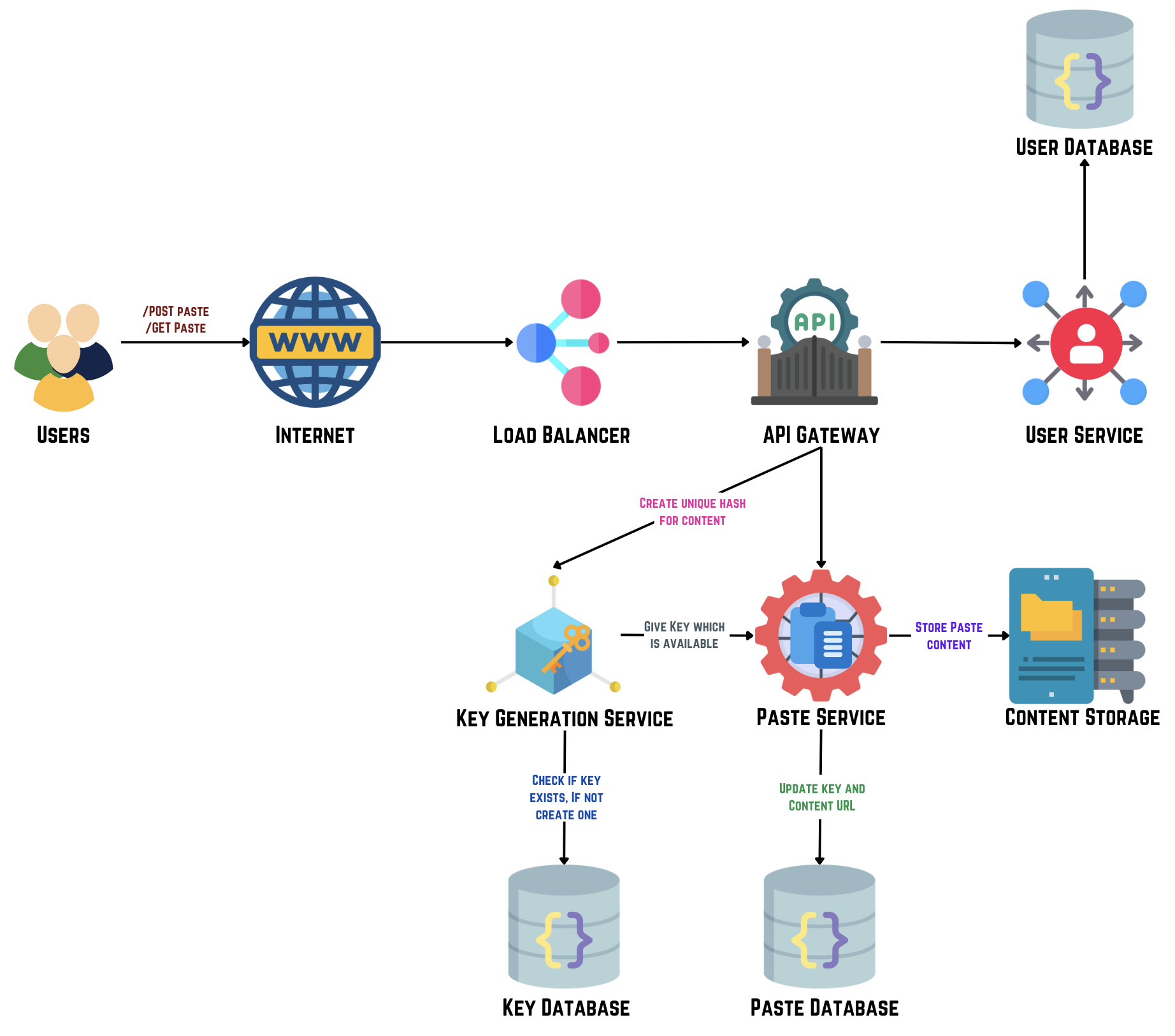
11. Cleanup Service
Clean Up Service plays a vital role in system maintenance, periodically scanning for and removing expired pastes. This helps manage storage space, maintain system performance, and ensure that temporary or time-sensitive content is appropriately handled.
High level architecture of Pastebin
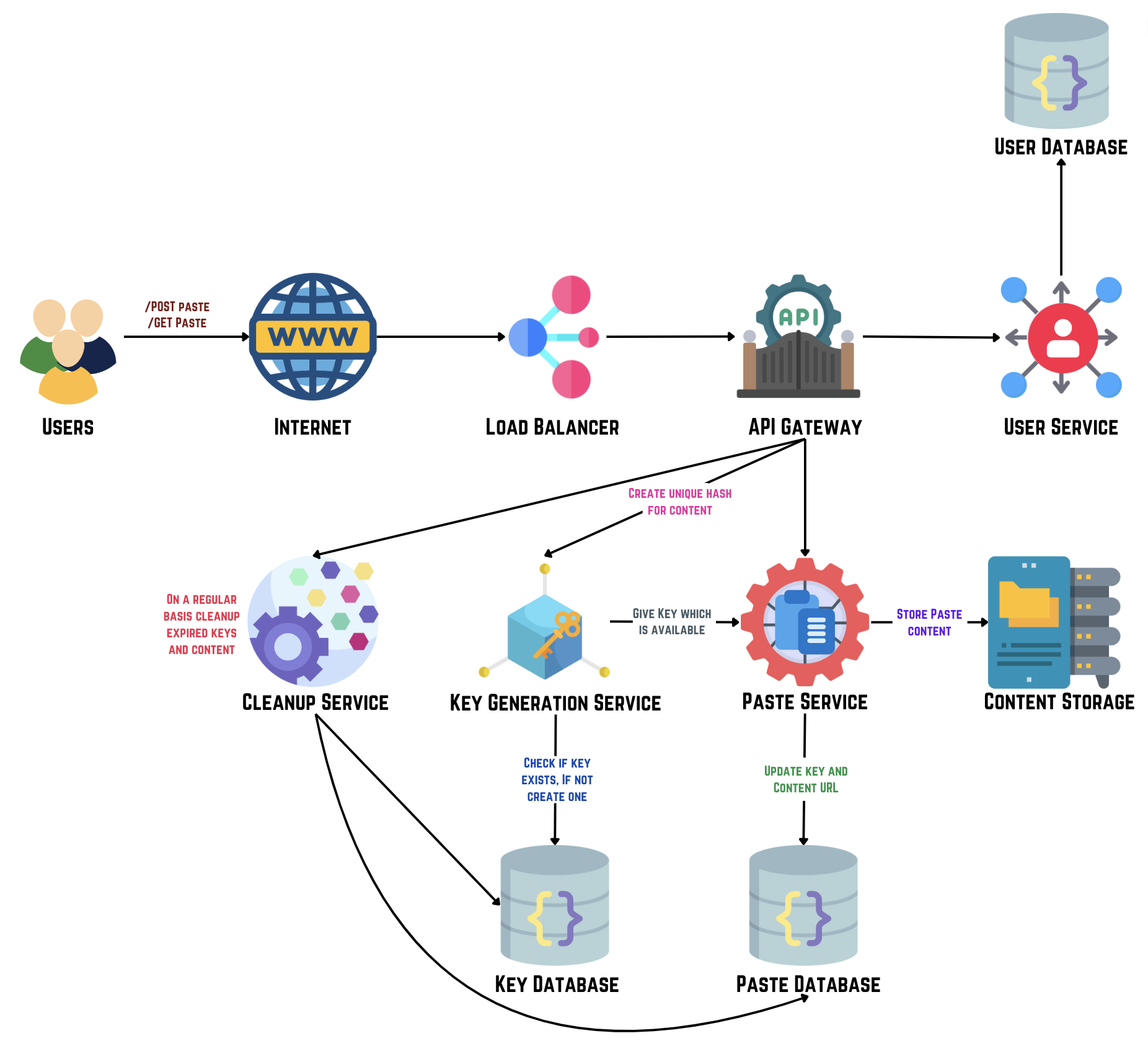
6. Futher Optimizations [2 - 5 mins]
1. Caching
Caching is crucial for optimizing performance and scalability in a service like Pastebin. By storing frequently accessed data such as text snippets, user metadata, and access logs in memory, caching reduces the need for repeated database queries. This enhances the speed of content retrieval and lightens the load on primary servers, allowing Pastebin to efficiently handle a large volume of concurrent users.
During peak usage periods or temporary network disruptions, caching ensures consistent service delivery by keeping essential data readily available. This reliability translates to a smoother user experience without interruptions. Overall, caching in systems like Redis, Memcached, and cloud-based solutions such as AWS ElastiCache and Microsoft Azure Cache for Redis is integral to Pastebin's infrastructure. These systems offer efficient key-value storage and scalable caching solutions, essential for managing Pastebin's dynamic content creation and sharing demands.
Data Stored in Cache
"key": "string",
"value": "string"
}
I. User Cache
The User Cache is an in-memory data store that holds frequently accessed user data to provide low-latency access. It is used to quickly retrieve user information without repeatedly querying the User Database.
Example User Cache
{
"key": "a152cda36954931db6c34c10964b2514",
"value": {
"user_id": "a152cda36954931db6c34c10964b2514",
"username": "testuser",
"email": "user@example.com",
"password_hash": "abe77298821adc672a57d84223479023",
"created_at": "2020-04-24 02:36:56"
}
},
{
...
},
]
II. Paste Cache
The User Cache is an in-memory data store that holds frequently accessed user data to provide low-latency access. It is used to quickly retrieve user information without repeatedly querying the User Database.
Example Paste Cache
{
"key": "082a2926b0c49f32870feb851c2e82e9",
"value": {
"paste_id": "082a2926b0c49f32870feb851c2e82e9",
"key_id": "137865431611218489155987613097308746794",
"content": "Hello There! Wanna try Pastebin?",
"visibility": "public",
"created_at": "2023-07-22 13:59:32"
}
},
{
...
},
]
High level architecture of Pastebin after Optimization
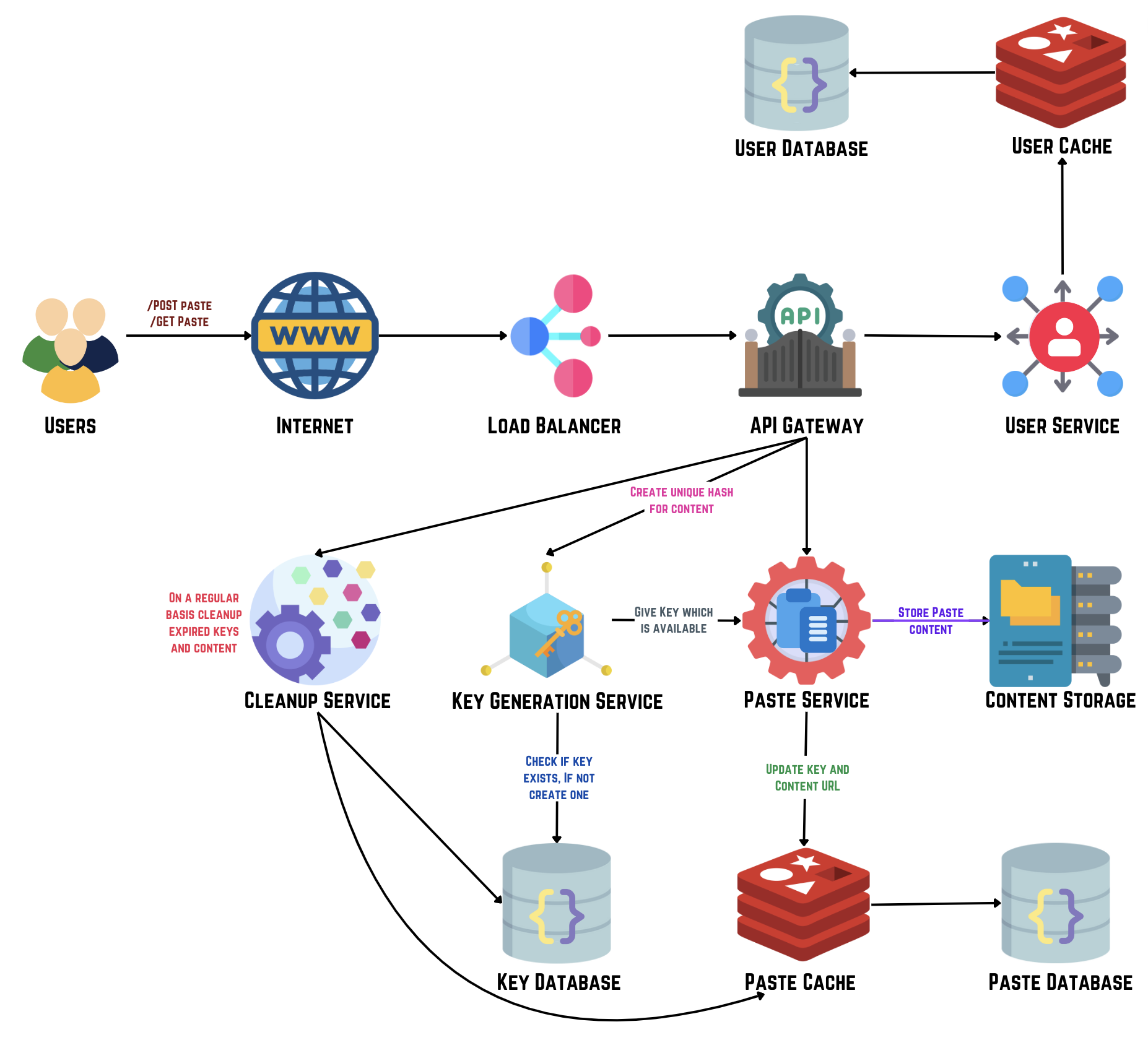
7. Data Flow [5-8 mins]
Posting a Paste:
- A user submits a paste through the Internet.
- The request hits the Load Balancer and is routed to the API Gateway.
- The API Gateway processes the /POST Paste request, uploading the content to the Content Storage.
- The paste metadata (e.g., paste_id, user_id, timestamp) is stored in the Paste Database.
- The Paste Cache may be updated for quick access to the newly created paste.
Getting a Paste:
- A user requests a paste through the Internet.
- The request goes through the Load Balancer to the API Gateway.
- The API Gateway processes the /GET Paste request by checking the Paste Cache.
- If the paste is not in the cache, it fetches it from the Paste Database.
- The content is retrieved from the Content Storage.
- The paste data is then served back to the user.
Don't forget to explain the end to end flow of your design!
This architecture is designed to be scalable, resilient, and efficient, ensuring that the platform can handle a high volume of user interactions and data processing with minimal latency and high availability.